
Hi Donald, suppose we built a SVC model in the online Jupyter Notebook, it can done as follows:
1. Save model to your library directory (refer to line #10-14)
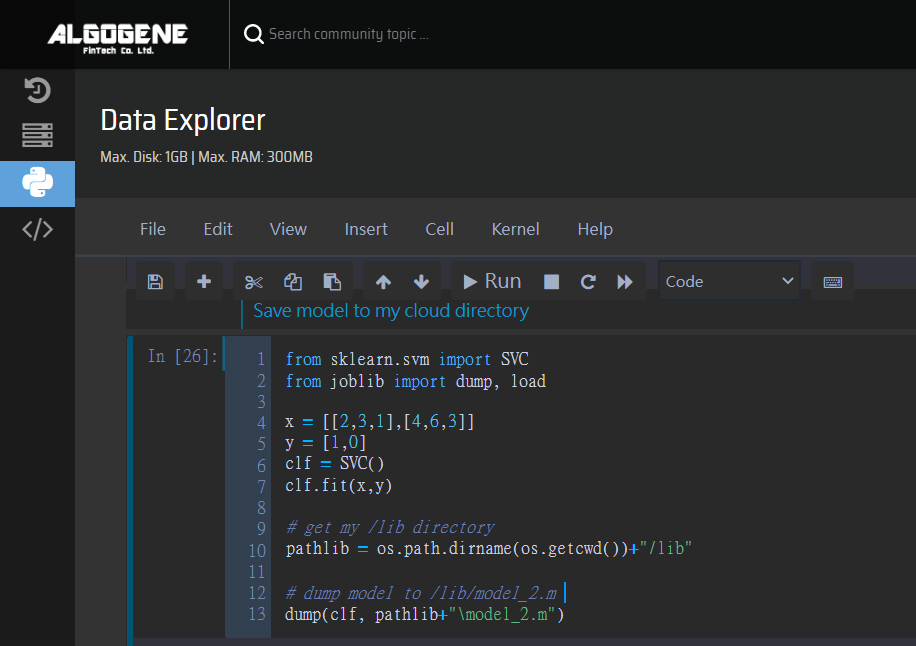
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | from sklearn.svm import SVC from joblib import dump, load import os x = [[2,3,1],[4,6,3]] y = [1,0] clf = SVC() clf.fit(x, y) # get my /lib directory pathlib = os.path.dirname(os.getcwd())+"/lib" # save model to /lib/model_2.m dump(clf, pathlib+"/model_2.m") |
2. Execute the code above, "model_2.m" will then be dumped to your cloud directory /lib.
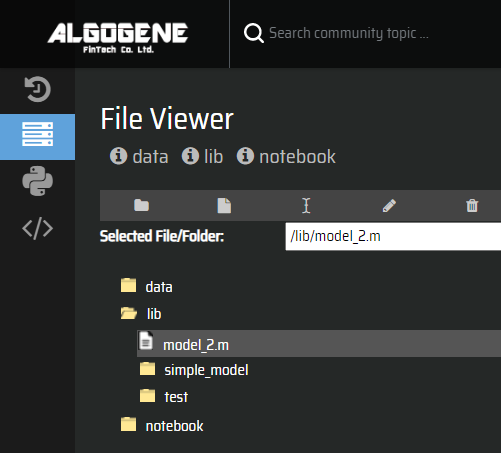
3. Go to "backtest", load your model from "self.evt.path_lib" (refer to line #15). After that, the model can be used for prediction throughout the backtest process (refer to line #23-25).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | from AlgoAPI import AlgoAPIUtil, AlgoAPI_Backtest from sklearn.svm import SVC from joblib import dump, load class AlgoEvent: def __init__(self): pass def start(self, mEvt): # start backtest self.evt = AlgoAPI_Backtest.AlgoEvtHandler(self, mEvt) # load my model_2.m self.model = joblib.load(self.evt.path_lib+"model_2.m") self.evt.start() def on_marketdatafeed(self, md, ab): # use self.model for new market data feed ... # model test dataset and print result x_test = [[1,2,3],[4,5,6]] result = self.model.predict(x_test) self.evt.consoleLog(result) pass |